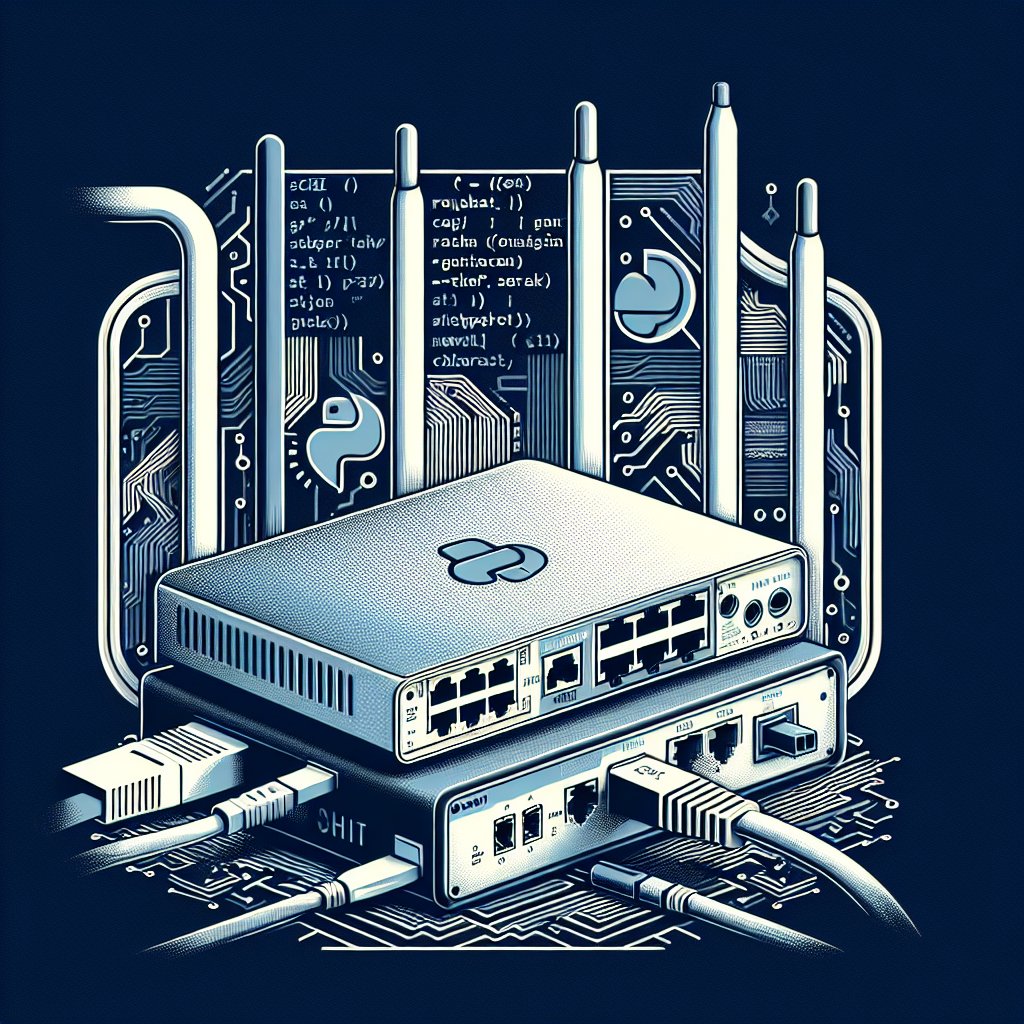
Mikrotik routers are powerful and versatile devices widely used in both home and enterprise environments. While the RouterOS interface is quite intuitive, automating configurations using Python can significantly streamline network management tasks. In this blog post, we’ll walk through how to connect to a Mikrotik router via SSH, configure basic settings, and retrieve essential network information using Python.
For this example, we assume the Mikrotik router is accessible at IP 192.168.88.1
, and the default username and password are admin
and password
, respectively.
Prerequisites
Before diving into the code, make sure you have the following prerequisites:
- A working Mikrotik router with RouterOS installed.
- Python installed on your machine.
- The
paramiko
library for SSH connections. You can install it using pip:bash
pip install paramiko
Establishing an SSH Connection
The first step in automating Mikrotik configurations is establishing an SSH connection. We’ll use the paramiko
library for this purpose. Here’s how you can establish a connection:
import paramiko
# Connection details
router_ip = "192.168.88.1"
username = "admin"
password = "password"
# Establish SSH connection
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
ssh.connect(router_ip, username=username, password=password)
print("Connected to the router")
This script connects to the router and prints a success message if the connection is established. It’s essential to handle any potential errors in a production environment, such as incorrect credentials or connection timeouts.
Configuring the Hostname
Once connected, you can send commands to configure the router. To change the router’s hostname, you can use the following script:
def set_hostname(ssh, hostname):
command = f"/system identity set name={hostname}"
stdin, stdout, stderr = ssh.exec_command(command)
if stderr.read():
print("Error setting hostname")
else:
print("Hostname set successfully")
set_hostname(ssh, "MyMikrotikRouter")
This function takes the SSH connection and the desired hostname as parameters, sends the command, and handles any errors that may occur.
Disabling Non-Essential IP Services
For security reasons, it’s crucial to disable non-essential services on the router. Here’s how you can do it:
def disable_services(ssh):
services = ["ftp", "telnet", "www", "api", "winbox", "api-ssl"]
for service in services:
command = f"/ip service set [find name={service}] disabled=yes"
ssh.exec_command(command)
print("Non-essential services disabled")
disable_services(ssh)
This script disables services like FTP, Telnet, and HTTP that are often unnecessary and can pose security risks if left enabled.
Disabling SIP ALG
The Session Initiation Protocol (SIP) ALG can sometimes interfere with VoIP traffic. Here’s how to disable it:
def disable_sip_alg(ssh):
command = "/ip firewall service-port set sip disabled=yes"
stdin, stdout, stderr = ssh.exec_command(command)
if stderr.read():
print("Error disabling SIP ALG")
else:
print("SIP ALG disabled successfully")
disable_sip_alg(ssh)
This command disables SIP ALG, ensuring that your VoIP traffic flows without interference.
Retrieving Network Information
Now that we’ve configured some basic settings, let’s retrieve essential network information like interfaces, IP addresses, and static routes.
Retrieving Interfaces:
def get_interfaces(ssh):
command = "/interface print"
stdin, stdout, stderr = ssh.exec_command(command)
interfaces = stdout.read().decode()
print("Interfaces:\n", interfaces)
get_interfaces(ssh)
Retrieving IP Addresses:
def get_ip_addresses(ssh):
command = "/ip address print"
stdin, stdout, stderr = ssh.exec_command(command)
ip_addresses = stdout.read().decode()
print("IP Addresses:\n", ip_addresses)
get_ip_addresses(ssh)
Retrieving Static Routes:
def get_static_routes(ssh):
command = "/ip route print"
stdin, stdout, stderr = ssh.exec_command(command)
routes = stdout.read().decode()
print("Static Routes:\n", routes)
get_static_routes(ssh)
These functions allow you to print out the current state of the router’s interfaces, IP addresses, and static routes.
Configuring IP Address on ether1
Next, let’s configure an IP address on the ether1
interface. This step is essential for establishing external connectivity:
def set_ip_address(ssh, interface, ip_address, subnet):
command = f"/ip address add address={ip_address}/{subnet} interface={interface}"
stdin, stdout, stderr = ssh.exec_command(command)
if stderr.read():
print(f"Error setting IP address on {interface}")
else:
print(f"IP address {ip_address}/{subnet} set on {interface} successfully")
set_ip_address(ssh, "ether1", "192.168.1.2", "24")
This function configures the specified IP address and subnet mask on the ether1
interface.
Configuring a Default Static Route
Finally, to ensure that the router can route traffic to the internet, we’ll configure a default static route:
def set_default_route(ssh, gateway):
command = f"/ip route add dst-address=0.0.0.0/0 gateway={gateway}"
stdin, stdout, stderr = ssh.exec_command(command)
if stderr.read():
print("Error setting default route")
else:
print("Default route set successfully")
set_default_route(ssh, "192.168.1.1")
This command adds a default route that directs all outbound traffic through the specified gateway.
Conclusion
Automating Mikrotik router configurations with Python can save time and reduce the likelihood of human error. In this post, we covered how to establish an SSH connection, configure the router’s hostname, disable non-essential services, and retrieve important network information. We also demonstrated how to configure an IP address and set a default static route. These basics provide a solid foundation for more complex automation tasks.
Feel free to expand on these examples to suit your specific network needs!